Safeguarding Personal Data in Sentiment Analysis: A Guide to PII Anonymization
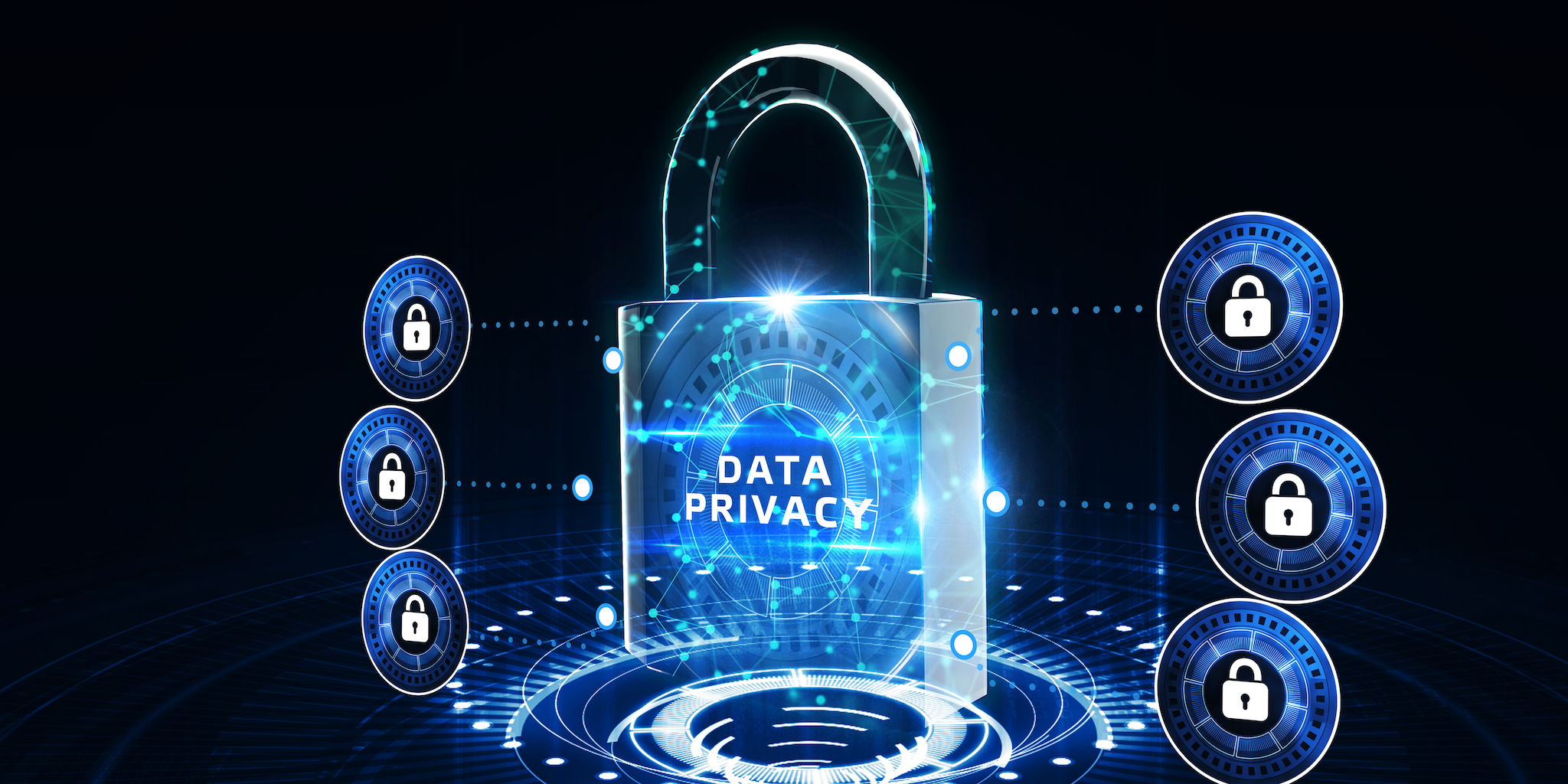
Let’s say you need to run sentiment analysis on a PII in dataset of reviews. You will use one of the pre-trained models offered by HuggingFace. There is only one problem: Your reviews contain names, addresses, and other Personal Identifiable Information (PII). What should you do? We can say what you shouldn’t do. Never send PII to a third-party API, even if they promise they won’t store the data. What can you do instead?
In our example, we will use a structured dataset with one column containing PII, but the same approach would also work if you were preparing data for an LLM.
Why shouldn’t you include PII in the dataset?
We don’t want to scare you, but here are some words that may bring you nightmares: GDPR, Law25, CCPA, LGPD, and, of course, the EU AI Act. Life would be too simple if those were all Data Protection Acts you must worry about. India, Australia, New Zealand, and Switzerland have their regulations too. The United Nations has 193 member states, so it’s almost certain there are some data protection regulations we never heard about. All of them are similar but also slightly different. Different just enough to cause legal problems when you are compliant with one but not the other. Suppose you remove PII before using the data to train a model or send the data to a third-party API. In that case, you will limit the areas where you must carefully check whether you comply with all relevant laws.
Also, most likely, the model will give you better results when you don’t include PII in dataset. Such improvement was observed by Sahil Verma, Michael Ernst, and Rene Just from the University of Washington in their research paper: “Removing biased data to improve fairness and accuracy” . In the paper, they noted the significance of removing the most biased data point (e.g., a loan application that was denied based on an indirect identifier like race). They removed the indirect identifier from the training data of the application that exhibited the most bias, ensuring that the model wouldn't be trained on it. However, they also compared their technique with the removal of sensitive identifiers that are considered "prohibited grounds" under human rights legislation. Their findings indicated that both techniques reduce bias to 0 and improve accuracy, although their method demonstrates a more significant improvement in accuracy compared to the removal of indirect discriminatory identifiers.
Should it matter for a sentiment analysis if the review’s author writes about Frieda or Emma? Perhaps having a model that sees names as relevant information and makes mistakes because of that isn’t a concern for you. And you may get accused of discrimination if your model always classifies reviews containing names popular in a minority group as negative.
In this text, we assume you are using a pre-trained third-party model. However, your users don’t care if you train the model yourself or use open-source models. The same rules often apply whether you send requests to HuggingFace API or remove PII from the training dataset while preparing your model.
What should you do?
If you remove PII in dataset, you won’t have any PII-related problems. Of course, removing PII isn’t as simple as it sounds. You have several options.
The easiest and the worst way would be to remove all rows containing any PII. Of course, it means you lose data that could be useful for training. What if all rows contain PII? Would you give up training the model? What if you are using a pre-trained model in production? Dropping requests in production won’t make anybody happy.
A better way is to replace all PII with a placeholder. Replace people’s names with the words “NAME GIVEN,” addresses with the word “LOCATION,” or phone numbers with the words “PHONE NUMBER.” Of course, nothing stops you from doing the replacement manually.
However, even if you outsource the work to hundreds of people, you still risk mistakes or data leaks. You can implement your data anonymization script based on Named Entity Recognition, but capturing the diversity of language and meticulously addressing corner case after corner case is no small feat. Private AI offers a faster and already tested method of removing PII from your data.
Using Private AI to remove PII before sending requests to HuggingFace API
We will process Starbucks reviews and use the twitter-roberta-base-sentiment-latest model to determine the sentiment of the reviews. We assume you have already installed the transformers library and loaded the data into a Pandas data frame. Right now, the dataset looks like this:
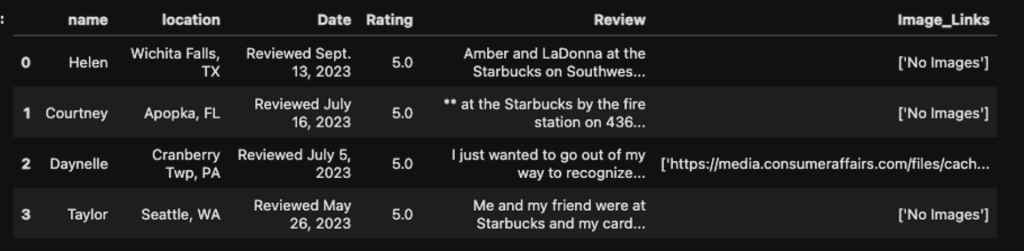
We already see two names of Starbucks employees and an address in the “Review” column.
We must decide which information to remove and what to keep. We don’t want to send people’s data to the HuggingFace API, but what about addresses? We have the “location” column, but a coffee shop address as a value isn’t precise enough on its own to uniquely re-identify an individual. It would be perfect to have the address of a location that gets lots of negative reviews if the review’s author included the address in their comment. Let’s remove people’s data but keep coffee shop addresses.
Before we start, we must install and initialize the Private AI client. For the sake of simplicity, we assume you have already loaded the Private AI API Key into the “api_key” variable and your HuggingFace token into the “hf_token” variable
!pip install privateai_client
from privateai_client import PAIClient
from privateai_client import request_objects
client = PAIClient(url="https://api.private-ai.com/deid/", api_key=api_key)
After initializing the client, we have to configure a request object and send it to Private AI. We will implement a function encapsulating those operations to use later in the Pandas apply function.
def redact_text(text):
proc_obj = request_objects.processed_text_obj(
type="MARKER",
pattern="BEST_ENTITY_TYPE"
)
entity_type_selector = request_objects.entity_type_selector_obj(
type="DISABLE",
value=[
'ORGANIZATION','LOCATION','LOCATION_ADDRESS',
'LOCATION_STATE','LOCATION_CITY','DATE'
]
)
entity_detection = request_objects.entity_detection_obj(
entity_types=[entity_type_selector]
)
text_req = request_objects.process_text_obj(
text=[text],
processed_text=proc_obj,
entity_detection=entity_detection
)
return client.process_text(text_req).processed_text[0]
The “processed_text_obj” function configures whether we care about individual entities in the text or a general category of personal data.
For example, setting the “pattern” parameter to “BEST_ENTITY_TYPE” gives us generic entity names such as “NAME_GIVEN”. If we set the parameter to UNIQUE_NUMBERED_ENTITY_TYPE, the first row of our example dataset would contain “NAME_GIVEN_1” and “NAME_GIVEN_2” after anonymization because the text includes the names of two people. The other available options are “ALL_ENTITY_TYPES” and “UNIQUE_HASHED_ENTITY_TYPE.” “ALL_ENTITY_TYPES” produces a hierarchy of entity types such as: “[NAME, NAME_GIVEN]”. The “UNIQUE_HASHED_ENTITY_TYPE” uses hashes to distinguish between entities instead of numbers, so the output could look like this: “NAME_GIVEN_q13R2.”
The number of people mentioned in a sentence seems irrelevant for sentiment analysis purposes, so we use the “BEST_ENTITY_TYPE” value.
Earlier, we decided to keep the location data in the reviews. Therefore, we have to configure an entity_type_selector and disable entity types related to locations. Similarly, the date in the review may be relevant information that we may need, so we disable the anonymization of dates. Note that, with external information like security camera footage of the date and location, it could be possible to narrow down the review to a small set of individuals. For this task, we assume the LLM provider does not have access to such external information.
We put the entity selector into an array and pass the selector to the entity_detection_obj to configure the redaction. We can pass multiple configurations at once! Afterward, we build a process_text_obj containing the configuration and the text we want to process.
In the last line, we send the request to the Private AI API and retrieve the redacted text.
When we use the function we have just defined, we get a dataset containing the original review text and the redacted version:
data_frame["redacted_review"] = data_frame["Review"].apply(redact_text)
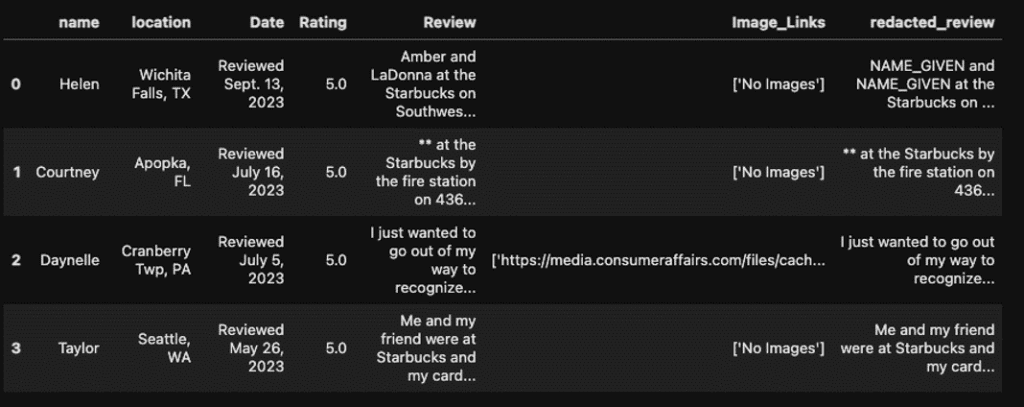
To use the review with anonymized PII, we implement the “get_sentiment” function. The function accepts the review text as a parameter, sends a request to the HuggingFace Inference API, and returns an array of scores for all supported sentiment labels.
import requests
def get_sentiment(text):
API_URL = "https://api-inference.huggingface.co/models/cardiffnlp/twitter-roberta-base-sentiment-latest"
headers = {"Authorization": f'Bearer {hf_token}'}
def query(payload):
response = requests.post(API_URL, headers=headers, json=payload)
return response.json()
output = query({
"inputs": text,
})
return output
Now, we send two requests to the API. The first one contains the PII, and the second one uses the redacted version.
print(get_sentiment(data_frame.iloc[0][4]))
print(get_sentiment(data_frame.iloc[0][6]))
As expected, we get similar scores in both cases:
[[{'label': 'positive', 'score': 0.9743512868881226}, {'label': 'neutral', 'score': 0.02006211131811142}, {'label': 'negative', 'score': 0.005586681421846151}]]
[[{'label': 'positive', 'score': 0.9751478433609009}, {'label': 'neutral', 'score': 0.019699547439813614}, {'label': 'negative', 'score': 0.005152557976543903}]]
Of course, in your production implementation, remember to use only the redacted column.
If you want to see the example code in a single Jupyter Notebook file, take a look at our Github repository.
By Bartosz Mikulski, Business Process Automation and AI Consultant